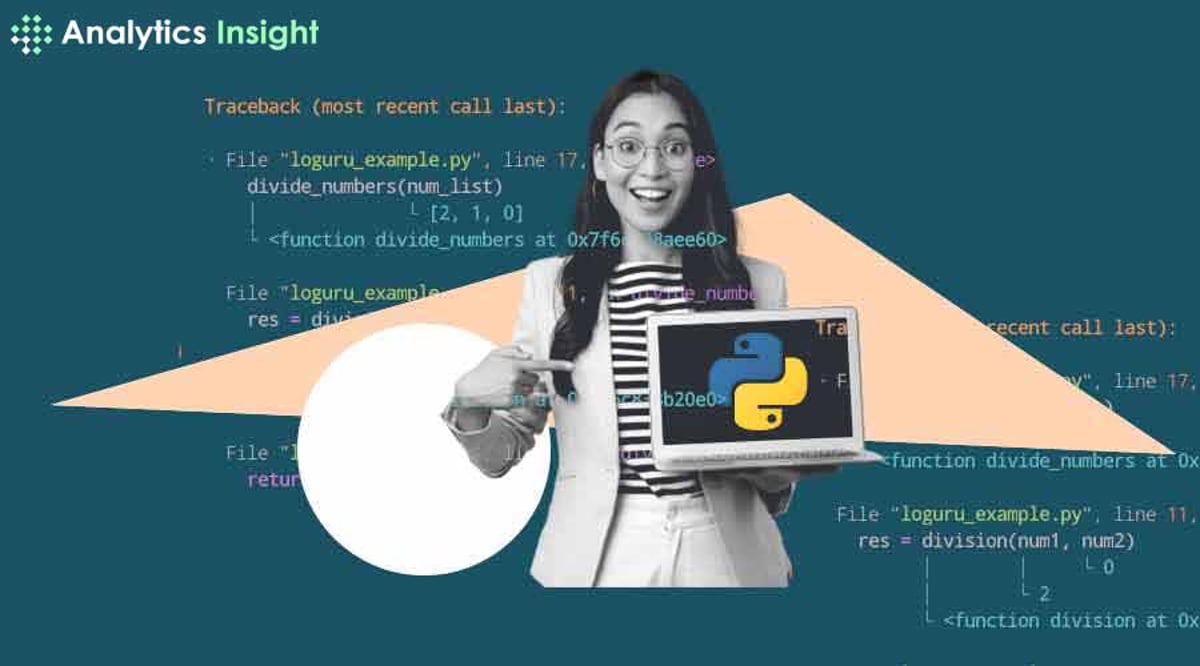
Python is one of the most versatile and beginner-friendly programming languages. Its simplicity and readability make it a favorite among new programmers, while its powerful libraries and frameworks have earned it a strong following in the professional community. Whether you’re new to programming or looking to deepen your expertise, building projects is the best way to enhance your skills. Here, we’ll explore the top 10 Python projects that will help you boost your coding skills, providing both a challenge and a way to showcase your abilities.
1. Personal Portfolio Website
Overview:
Creating a personal portfolio website is a perfect beginner project that allows you to showcase your skills while learning the fundamentals of web development. A portfolio website can include sections like your bio, projects, skills, and a contact form. This project will introduce you to front-end technologies like HTML, CSS, and JavaScript, alongside Python’s Flask or Django frameworks for the back end.
Skills Learned:
- Web Development: Learn the basics of web development with HTML, CSS, and JavaScript.
- Backend Development: Understand the fundamentals of Flask or Django for handling the server side.
- Routing and Templating: Use Jinja2 templates in Flask/Django to create dynamic web pages.
- Deployment: Deploy your website using platforms like Heroku or PythonAnywhere.
Resources:
2. Weather Forecasting App
Overview:
Building a weather forecasting app is a great way to learn how to interact with APIs (Application Programming Interfaces) and manage data. The app can fetch weather data for any location using a weather API, such as OpenWeatherMap. You can build a simple command-line tool or a full-fledged web app with a user-friendly interface.
Skills Learned:
- API Integration: Learn how to interact with external APIs to fetch data.
- JSON Parsing: Understand how to parse and manipulate JSON data in Python.
- Error Handling: Improve your skills in managing exceptions and ensuring your app handles errors gracefully.
- User Interface Design: If you opt for a web app, enhance your UI/UX design skills.
Resources:
- OpenWeatherMap API: OpenWeather
3. Expense Tracker
Overview:
An expense tracker is a practical project that allows you to manage and track your expenses. You can build this as a simple command-line application or a more complex web app with a database to store transaction records. Features might include adding, viewing, and categorizing expenses, as well as generating reports or visualizing spending habits over time.
Skills Learned:
- Database Management: Learn how to use SQLite or PostgreSQL for storing and retrieving data.
- Data Visualization: Utilize libraries like Matplotlib or Seaborn to create charts and graphs that visualize spending.
- CRUD Operations: Master Create, Read, Update, and Delete operations on data.
- File Handling: Work with CSV files for importing/exporting data.
Resources:
- SQLite Documentation: SQLite
- Matplotlib Documentation: Matplotlib
4. Chat Application
Overview:
Building a chat application can be a challenging yet rewarding project. The application can include features such as real-time messaging, user authentication, and group chats. This project will give you experience with socket programming, threading, and network protocols, and is an excellent introduction to real-time communication systems.
Skills Learned:
- Socket Programming: Understand the basics of socket programming for real-time communication.
- Multithreading: Learn how to handle multiple connections simultaneously using threading.
- User Authentication: Implement user login and registration systems.
- Frontend Development: Build a simple GUI with Tkinter or use Flask/Django for a web-based chat.
Resources:
5. To-Do List Application
Overview:
A to-do list app is a straightforward project but offers ample room for learning and improvement. The app allows users to create, update, delete, and manage tasks. You can start with a basic version and progressively add more features, such as setting deadlines, categorizing tasks, and sending notifications.
Skills Learned:
- Project Structuring: Understand how to structure a small-to-medium sized project.
- Database Interaction: Manage task data using SQLite or another database.
- Time Management: Incorporate date and time features for deadlines.
- UI/UX: Develop a clean, user-friendly interface using Tkinter, PyQt, or web technologies.
Resources:
- PyQt5 Documentation: PyQt5
- Tkinter Tutorials: Real Python

6. Blog Platform
Overview:
Creating a blogging platform is a comprehensive project that can significantly enhance your understanding of web development. This project involves creating user accounts, allowing users to create and edit posts, commenting on posts, and categorizing content by tags. It’s a more complex version of a content management system (CMS) and is best suited for those who have a grasp of basic web development.
Skills Learned:
- Full-Stack Development: Work on both frontend and backend aspects of a web application.
- Database Models: Learn about relational databases and ORM (Object-Relational Mapping) with Django or SQLAlchemy.
- User Authentication: Implement secure user login, registration, and session management.
- Content Management: Manage dynamic content, such as blog posts, categories, and comments.
Resources:
- Django Documentation: Django
- Flask-SQLAlchemy Documentation: SQLAlchemy
7. E-commerce Website
Overview:
An e-commerce website project is a more advanced challenge that can demonstrate your ability to build complex systems. This project includes features like product listings, shopping carts, user authentication, and payment processing. It’s a great way to learn about the full-stack development lifecycle and how different components of a web application interact.
Skills Learned:
- Advanced Web Development: Gain experience in developing robust, scalable web applications.
- Payment Gateway Integration: Learn how to integrate third-party payment services like Stripe or PayPal.
- Inventory Management: Implement systems for managing product inventories, pricing, and categories.
- Security: Ensure the application is secure against common vulnerabilities.
Resources:
8. Automation Scripts
Overview:
Automation scripts are small Python programs designed to automate repetitive tasks. Examples include scripts to automate file organization, send automated emails, scrape data from websites, or automate data entry tasks. This project is excellent for improving your understanding of the Python Standard Library and learning how to write efficient, reusable code.
Skills Learned:
- Web Scraping: Learn how to extract data from websites using libraries like BeautifulSoup or Scrapy.
- File Handling: Automate tasks such as file renaming, moving, or copying.
- Regular Expressions: Use regex to search and manipulate strings.
- Task Scheduling: Automate recurring tasks using schedulers like Cron or Windows Task Scheduler.
Resources:
- BeautifulSoup Documentation: BeautifulSoup
- Python’s
re
module documentation: Regex
9. Machine Learning Model
Overview:
Building a machine learning model is an advanced project that introduces you to data science and AI. You can choose a problem like sentiment analysis, image recognition, or predictive modeling. This project involves data collection, data preprocessing, model training, and evaluation, and it’s an excellent way to get hands-on experience with libraries like TensorFlow, Keras, or Scikit-learn.
Skills Learned:
- Data Preprocessing: Learn how to clean and prepare data for analysis.
- Model Building: Build and train machine learning models using popular libraries.
- Evaluation Metrics: Understand how to evaluate the performance of your models using accuracy, precision, recall, etc.
- Model Deployment: Deploy your model to a web app or as an API for real-world use.
Resources:
- Scikit-learn Documentation: Scikit-learn
- TensorFlow Documentation: TensorFlow
10. Game Development
Overview:
Developing a game in Python is a fun way to learn programming concepts while applying your creativity. You can start with simple games like Tic-Tac-Toe, Snake, or even a text-based adventure game. As you progress, you can build more complex games with graphics using libraries like Pygame.
Skills Learned:
- Game Logic: Understand how to implement game rules and mechanics.
- Graphics Programming: Learn how to use Pygame for rendering graphics, handling events, and managing game states.
- Object-Oriented Programming: Use OOP principles to structure your game’s code.
- Animation: Create smooth animations and transitions in your game.